Custom Inputs
Here are the examples of custom inputs made with the help of MUI's Radio and Checkbox. Please refer to our docs for more details.
Basic
Free
Get 1 project with 1 team member.
Premium
$5.00
Get 5 projects with 5 team members.
Discount
20%
Wow! Get 20% off on your next purchase!
Updates
Free
Get Updates regarding related products.
Starter
A simple start for everyone.
Standard
For small to medium businesses.
Enterprise
Solution for big organizations.
Backup
Backup every file from your project.
Encrypt
Translate your data to encrypted text.
Site Lock
Security tool to protect your website.
Starter
A simple start for everyone.
Standard
For small to medium businesses.
Enterprise
Solution for big organizations.
Backup
Backup every file from your project.
Encrypt
Translate your data to encrypted text.
Site Lock
Security tool to protect your website.
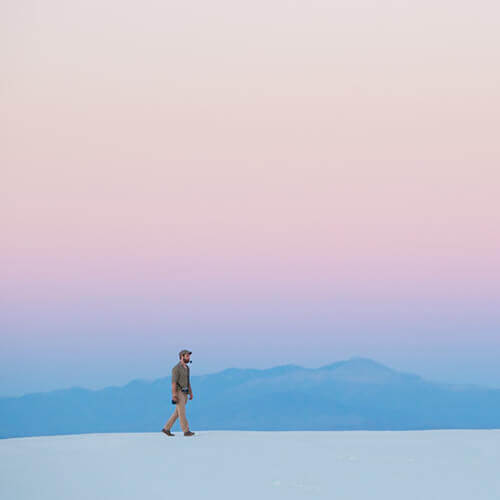
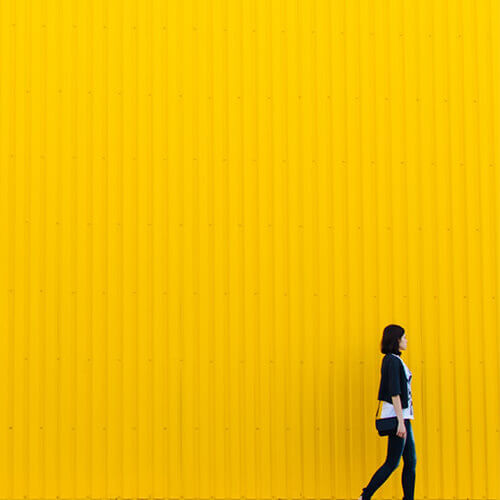
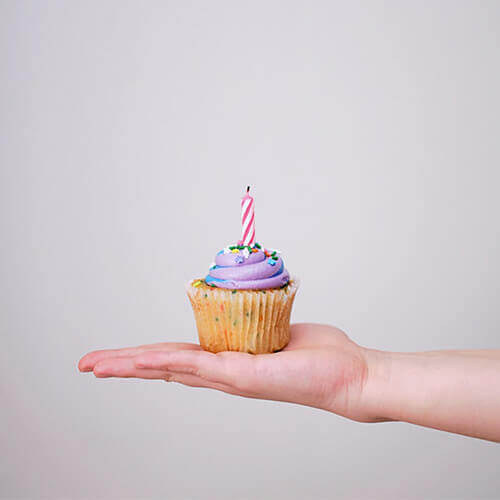
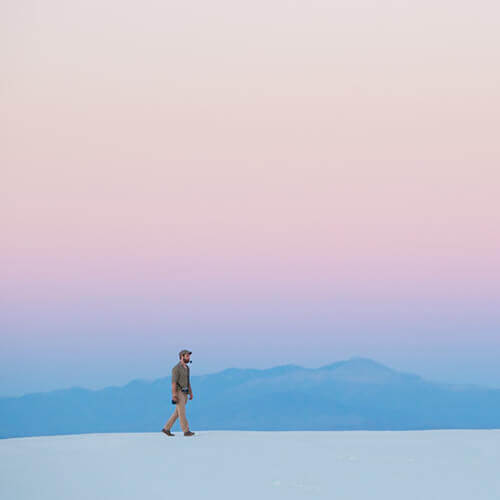
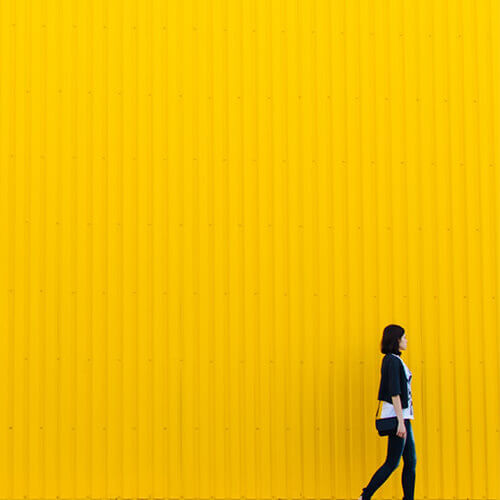
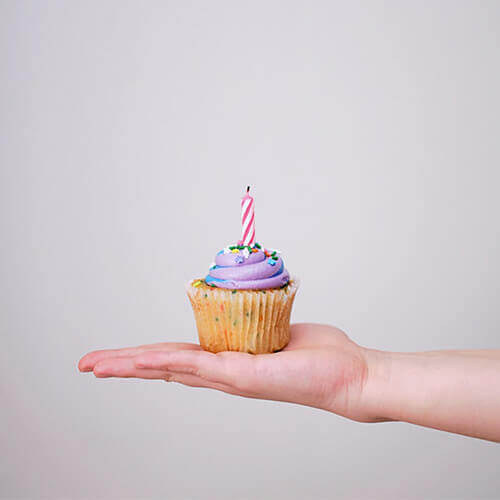