# Layout Overrides
# Overview
The src/layouts
folder in the root of the src
folder is for users to override layouts built in the src/@core
folder. It is highly recommended to override layouts in the src/layouts
folder instead of directly working in the src/@core
folder. This will ease your updates to newer versions without facing any hassle of backing up your current code, else it will override your changes in the src/@core
folder each time you take an update.
Most of the layout components explained on this page are overridden in the src/layouts/UserLayout.tsx
file.
# Layout PropTypes
Following is the PropTypes for Vertical and Horizontal Layouts only.
export type LayoutProps = {
hidden: boolean
settings: Settings
children: ReactNode
scrollToTop?: (props?: any) => ReactNode
saveSettings: (values: Settings) => void
footerProps?: {
sx?: SxProps<Theme>
content?: (props?: any) => ReactNode
}
horizontalLayoutProps?: {
appBar?: {
componentProps?: AppBarProps
content?: (props?: any) => ReactNode
branding?: (props?: any) => ReactNode
}
navMenu?: {
sx?: SxProps<Theme>
navItems?: HorizontalNavItemsType
content?: (props?: any) => ReactNode
}
}
verticalLayoutProps: {
appBar?: {
componentProps?: AppBarProps
content?: (props?: any) => ReactNode
}
navMenu: {
lockedIcon?: ReactNode
unlockedIcon?: ReactNode
navItems?: VerticalNavItemsType
content?: (props?: any) => ReactNode
branding?: (props?: any) => ReactNode
afterContent?: (props?: any) => ReactNode
beforeContent?: (props?: any) => ReactNode
componentProps?: Omit<SwipeableDrawerProps, 'open' | 'onOpen' | 'onClose'>
}
}
}
# Vertical Layout
You can override the following layout components:
- App Logo
- Menu collapse icons
- Menu content
- Add content before menu items
- Add content after menu items
- Hide menu based on screen size
- Navbar (or AppBar) Content
- Footer content
- How to add custom styles
# 1. App Logo
If you want to change the app logo, you need to use the verticalLayoutProps
prop with the Layout
component and the type accepted by this prop is:
verticalLayoutProps: {
navMenu: {
branding?: (props?: any) => ReactNode
}
}
Here is the code to change the app logo:
Result:
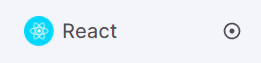
NOTE
When you override the app logo and when the menu is collapsed, padding-left
of the menu header will reduce to 0. To center align your logo, you need to manually add margin-left
to your overridden logo.
# 2. Menu collapse icons
If you want to change the icons for collapsing the vertical menu, you need to use the verticalLayoutProps
prop with the Layout
component and the type accepted by this prop is:
verticalLayoutProps: {
navMenu: {
lockedIcon?: ReactNode
unlockedIcon?: ReactNode
}
}
Here is the code to change the icons for collapsing the vertical menu:
Result of lockedIcon
:
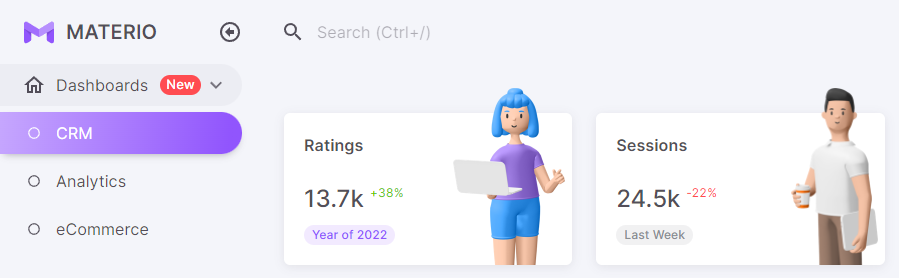
Result of unlockedIcon
:
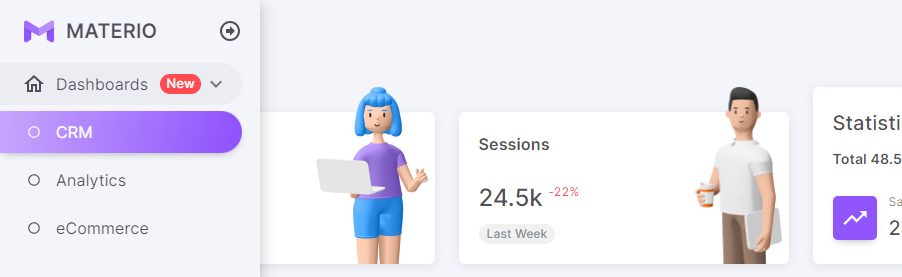
# 3. Menu content
If you want to change the menu content, you need to use the verticalLayoutProps
prop with the Layout
component and the type accepted by this prop is:
verticalLayoutProps: {
navMenu: {
content?: (props?: any) => ReactNode
}
}
Here is the code to change the menu content:
Result:
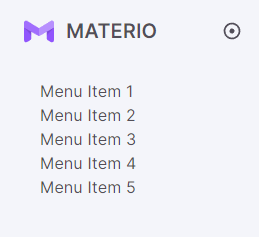
# 4. Add content before menu items
If you want to add something before the menu items, you need to use the verticalLayoutProps
prop with the Layout
component and the type accepted by this prop is:
verticalLayoutProps: {
navMenu: {
beforeContent?: (props?: any) => ReactNode
}
}
Here is the code to add user info before the menu items:
Result:
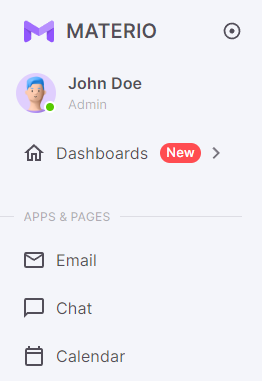
# 5. Add content after menu items
If you want to add something after the menu items, you need to use the verticalLayoutProps
prop with the Layout
component and the type accepted by this prop is:
verticalLayoutProps: {
navMenu: {
afterContent?: (props?: any) => ReactNode
}
}
Here is the code to add menu footer info after the menu items:
Result:
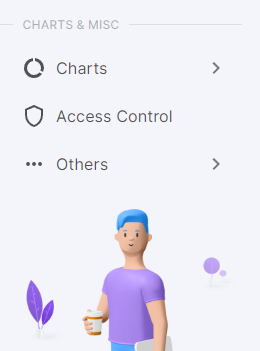
# 6. Hide menu based on screen size
The hidden
prop is used to hide the vertical menu at a given screen size. The menu will only be accessible from the Hamburger menu icon which is known as the Vertical Overlay Menu. You can change the screen size from which you want to hide the vertical menu.
In the example below, the vertical menu is visible above the lg
breakpoint and on screen size below the lg
breakpoint, it will change to the vertical overlay menu which can be accessed from the Hamburger menu icon.
const hidden = useMediaQuery((theme: Theme) => theme.breakpoints.down('lg'))
You can also change its value using a specific screen size:
const hidden = useMediaQuery('(max-width:1365px)')
# 7. Navbar (or AppBar) Content
The content in the appBar comes from the user side itself and thus, it would be very easy and convenient for you to change anything in the appBar. You just have to change the code in the src/layouts/components/vertical/AppBarContent.tsx
file as per your requirements. The appBar component is then passed in the verticalLayoutProps
prop with the Layout
component and the type accepted by this prop is:
verticalLayoutProps: {
appBar?: {
content?: (props?: any) => ReactNode
}
}
Here is the code to change the appBar:
Result:

# 8. Footer content
If you want to change the footer content, you need to use the footerProps
prop with the Layout
component and the type accepted by this prop is:
footerProps?: {
content?: (props?: any) => ReactNode
}
Here is the code to change the footer content:
Result:

# 9. How to add custom styles
You can add your custom styles for the whole layout with the help of footerProps
and verticalLayoutProps
props with the Layout
component. The type for the same is:
footerProps?: {
sx?: SxProps<Theme>
}
verticalLayoutProps: {
appBar?: {
componentProps?: AppBarProps
}
navMenu: {
componentProps?: Omit<SwipeableDrawerProps, 'open' | 'onOpen' | 'onClose'>
}
}
Refer to the following example for adding your custom styles:
# Horizontal Layout
Important!
As mentioned here, you need to add all the necessary items for the Vertical Layout. Please follow these steps to add items for the Vertical Layout and then follow the steps explained below.
You can override the following layout components:
- App Logo
- Menu content
- Hide menu based on screen size
- AppBar Content
- Footer content
- How to add custom styles
# 1. App Logo
If you want to change the app logo, you need to use the verticalLayoutProps
and horizontalLayoutProps
props with the Layout
component and the type accepted by these props is:
verticalLayoutProps: {
navMenu: {
branding?: (props?: any) => ReactNode
}
}
horizontalLayoutProps?: {
appBar?: {
branding?: (props?: any) => ReactNode
}
}
Here is the code to change the app logo:
Result:
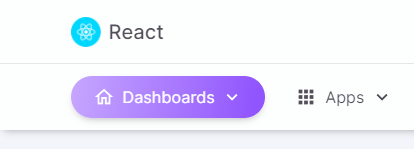
# 2. Menu content
If you want to change the menu content, you need to use the verticalLayoutProps
and horizontalLayoutProps
props with the Layout
component and the type accepted by these props is:
verticalLayoutProps: {
navMenu: {
content?: (props?: any) => ReactNode
}
}
horizontalLayoutProps?: {
navMenu: {
content?: (props?: any) => ReactNode
}
}
Here is the code to change the menu content:
Result:

# 3. Hide menu based on screen size
The hidden
prop is used to hide the horizontal menu at a given screen size. The menu will only be accessible from the Hamburger menu icon which is known as the Vertical Overlay Menu. You can change the screen size from which you want to hide the horizontal menu.
In the example below, the horizontal menu is visible above the lg
breakpoint and on screen size below the lg
breakpoint, it will change to the vertical overlay menu which can be accessed from the Hamburger menu icon.
const hidden = useMediaQuery((theme: Theme) => theme.breakpoints.down('lg'))
You can also change its value using a specific screen size:
const hidden = useMediaQuery('(max-width:1365px)')
# 4. AppBar Content
The content in the appBar which is on the right side comes from the user side itself and thus, it would be very easy and convenient for you to change anything in the appBar. You just have to change the code in the src/layouts/components/horizontal/AppBarContent.tsx
file. The appBar component is then passed in the horizontalLayoutProps
prop with the Layout
component. The appBar component for the Vertical Layout (which comes from the src/layouts/components/vertical/AppBarContent.tsx
file) is passed in the verticalLayoutProps
prop with the Layout
component.
The type accepted by these props is:
verticalLayoutProps: {
appBar?: {
content?: (props?: any) => ReactNode
}
}
horizontalLayoutProps?: {
appBar?: {
content?: (props?: any) => ReactNode
}
}
Suppose you need the app logo, navigation menu as well as some actions in one line, then you need to follow the steps given below.
Firstly, you need to hide the navigation menu section which is below the appBar from the src/configs/themeConfig.ts
file:
Then you need to change the appBar accordingly:
Then you need to pass that component in the horizontalLayoutProps
prop with the Layout
component:
Result:

# 5. Footer content
If you want to change the footer content, you need to use the footerProps
prop with the Layout
component and the type accepted by this prop is:
footerProps?: {
content?: (props?: any) => ReactNode
}
Here is the code to change the footer content:
Result:

# 6. How to add custom styles
You can add your custom styles for the whole layout with the help of footerProps
, verticalLayoutProps
and horizontalLayoutProps
props with the Layout
component. The type for the same is:
footerProps?: {
sx?: SxProps<Theme>
}
verticalLayoutProps: {
appBar?: {
componentProps?: AppBarProps
}
navMenu: {
componentProps?: Omit<SwipeableDrawerProps, 'open' | 'onOpen' | 'onClose'>
}
}
horizontalLayoutProps?: {
appBar?: {
componentProps?: AppBarProps
}
navMenu?: {
sx?: SxProps<Theme>
}
}
Refer to the following example for adding your custom styles:
# Blank Layout
If you want to change the Blank Layout, you need to follow these steps:
- Make a new file (let us say
UserBlankLayout.tsx
file name) in thesrc/layouts
folder - Copy the whole code from the
src/@core/layouts/BlankLayout.tsx
file and paste it into thesrc/layouts/UserBlankLayout.tsx
file - Edit the
src/layouts/UserBlankLayout.tsx
file as per your requirements - Now, to use this layout in any of your pages, you need to do this:
# Blank Layout with AppBar
If you want to change the navbar, you need to follow these steps:
- Make a new file (let us say
UserBlankLayoutWithAppBar.tsx
file name) in thesrc/layouts
folder - Copy the whole code from the
src/@core/layouts/BlankLayoutWithAppBar.tsx
file and paste it into thesrc/layouts/UserBlankLayoutWithAppBar.tsx
file - Edit the
src/layouts/UserBlankLayoutWithAppBar.tsx
file as per your requirements - Now, to use this layout in any of your pages, you need to do this:
# Scroll to Top
If you want to change the scroll to top component, you need to use the scrollToTop
prop with the Layout
component in the src/layouts/UserLayout.tsx
file and the type accepted by this prop is:
scrollToTop?: (props?: any) => ReactNode
Here is the code to change the scroll to top component:
Result:
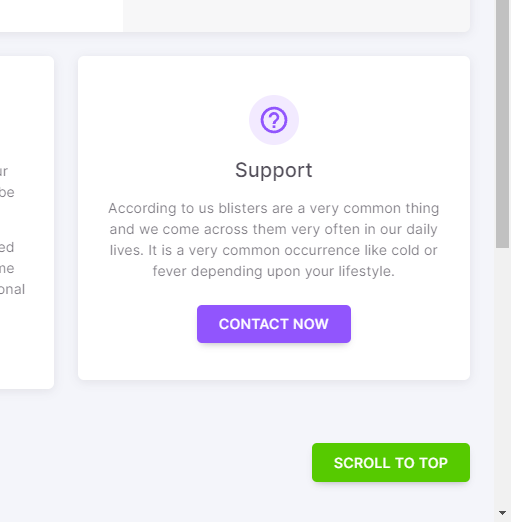
# Customizer
If you want to override the customizer or make a custom customizer, then follow these steps:
- Hide the customizer from the
src/configs/themeConfig.ts
file
- Make a new file (let us say
Customizer.tsx
file name) in thesrc/layouts/components
folder - Copy the whole code from the
src/@core/components/customizer/index.tsx
file and paste it into thesrc/layouts/components/Customizer.tsx
file - Edit the
src/layouts/components/Customizer.tsx
file as per your requirements - Render this customizer in the
src/layouts/UserLayout.tsx
file: