Badges
Please refer to MUI's official docs for more details on component's usage guide and API documentation.
Basic Badges
Use badgeContent
prop for the text inside the badge and color
prop for different colors of a badge.
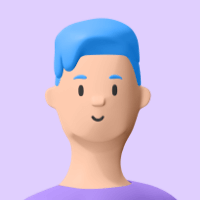
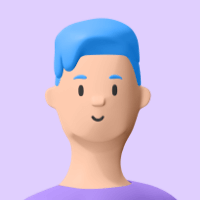
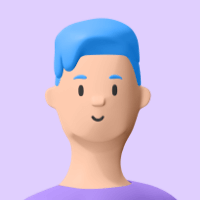
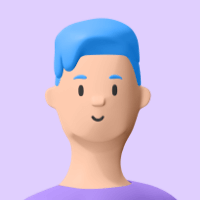
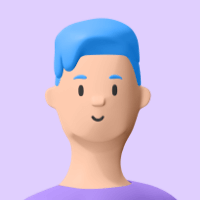
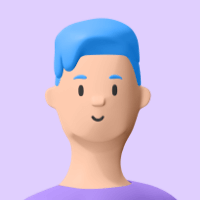
Dot Badges
Use variant='dot'
prop for dot badges.
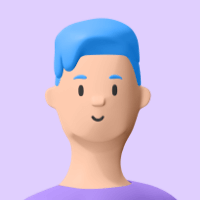
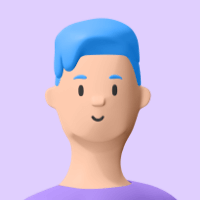
Typography
Badge Alignment
Use anchorOrigin
prop to move the badge to any corner of the wrapped element.
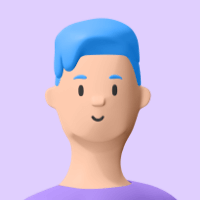
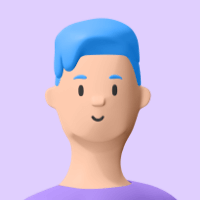
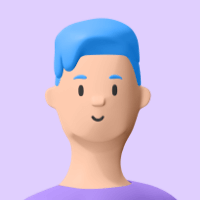
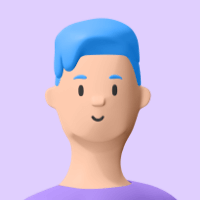
Maximum Value
Use max
prop to cap the value of the badge content.
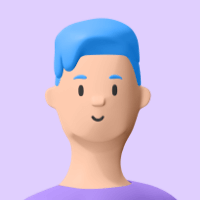
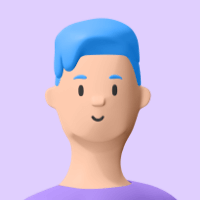
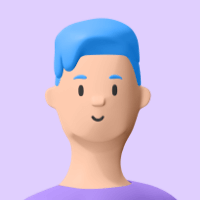
Badge Overlap
Use overlap
prop to place the badge relative to the corner of the wrapped element.
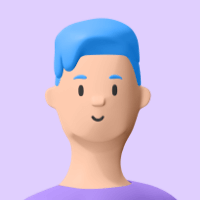
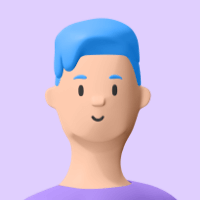
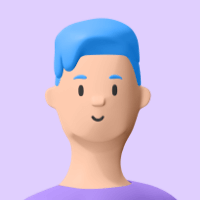
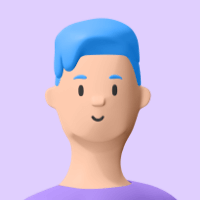
Badge visibility
The visibility of badges can be controlled using invisible
prop.
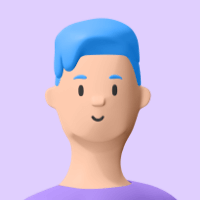
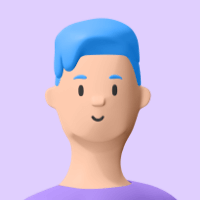
Custom Tonal Badges
If you want to use Tonal variant of the badges, you need to use our custom component with tonal='true'
prop.
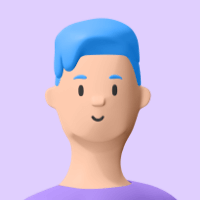
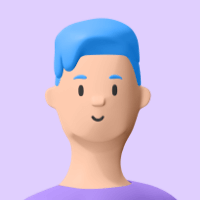
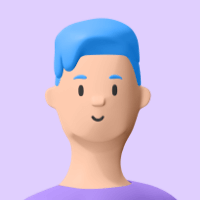
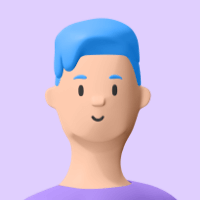
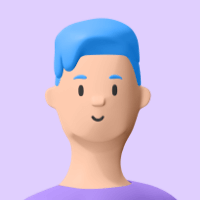
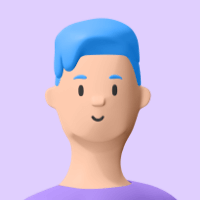